【基本】Pythonの数値型の宣言と色々な計算方法について解説!
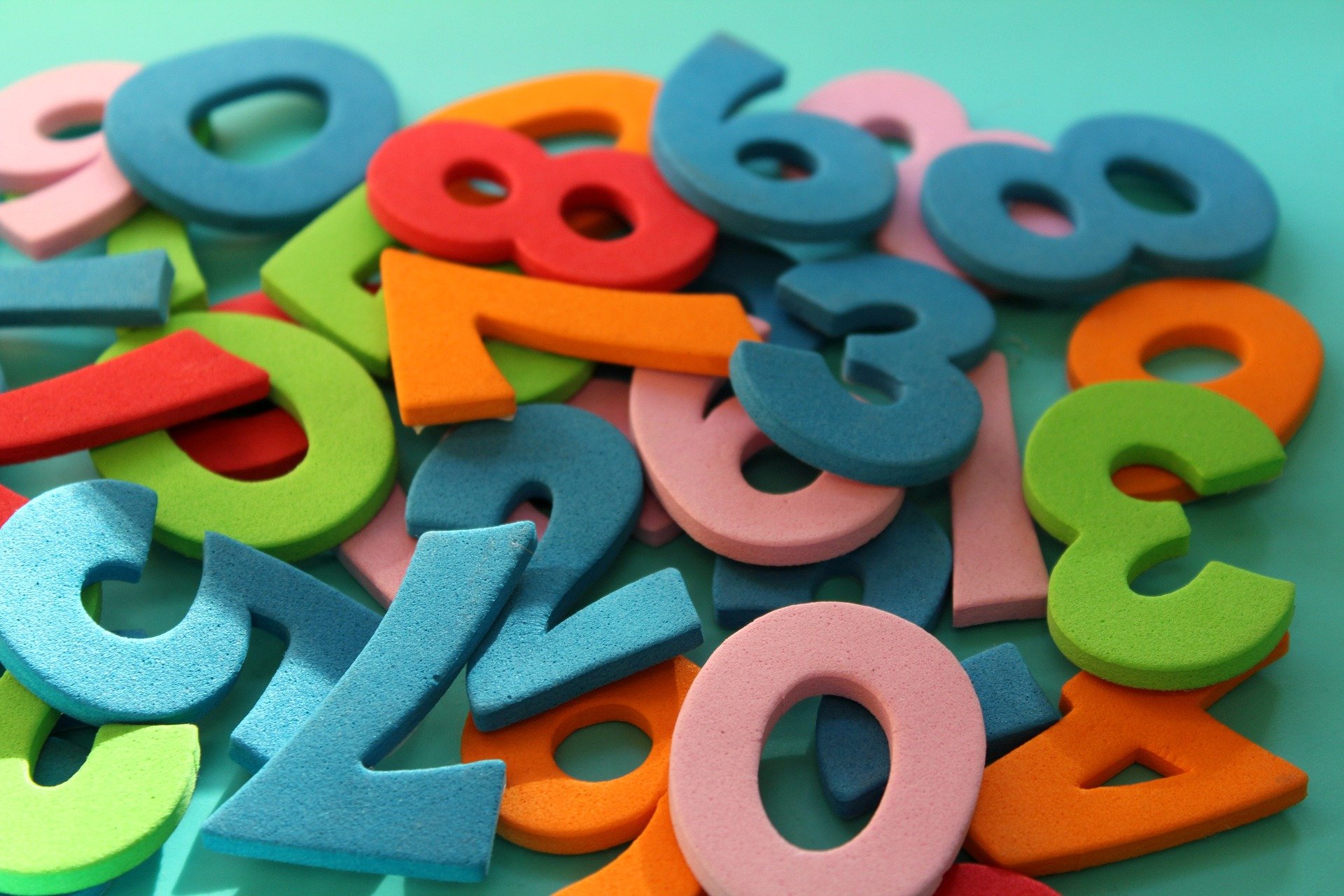
こんにちは、爽です。皆さん、いかがお過ごしでしょうか?
今回はPythonの数値型の宣言と数値型の色々な計算方法について確認します。
数値型の操作はプログラミングの基本ともなりますので、ぜひ覚えて頂ければと思います。
■この記事の対象読者
・Pythonに興味がある方
・Pythonを学び始めてみたい方
・Pythonでどんな計算ができるのか知りたい方
なお、私はPythonをAnacondaをインストールしてJupyterで実行しています。MacのAnacondaのインストール方法とJupyterの使い方は下記記事にまとめているので良かったらご参考ください。
それではどうぞ!
数値型の宣言方法
数値型には整数型と浮動小数点型の2種類があります。
整数型の宣言とデータ型の確認
整数型とはint型のことで、int型は変数に整数を代入することで宣言できます。
#整数型
num = 1
num
# 1
#変数のデータ型確認
type(num)
# int
浮動小数点型の宣言とデータ型の確認
続いて浮動小数点型とはfloat型のことで、float型は変数に小数点を含む数値を代入することで宣言できます。
#浮動小数点型
PI = 3.14
PI
# 3.14
#変数のデータ型確認
type(PI)
# float
このようにPythonでは変数名の前にデータ型を宣言する必要がないので、コードがスッキリするのがわかると思います。
Pythonのデータ型を指定した変数宣言
ちなみにPythonでは「変数名:データ型」の書式でデータ型を指定して変数宣言をすることもできますが、一般的ではありません。
#データ型の指定もできるが、あまり一般的ではない
num :int = 1
#変数のデータ型確認
type(num)
# int
数値型の計算方法
さて、続いて数値型の計算方法について確認します。
四則演算(和、差、積、商)
まずは簡単な四則演算で、これは直感的に分かるものが多いです。
#四則演算
#変数宣言
num = 4
value = 2
#足し算は"+"(プラス)を使用
num + value
# 6
#引き算は"-"(ハイフン)を使用
num - value
# 2
#掛け算は"*"(アスタリスク)を使用
num * value
# 8
#割り算は"/"(スラッシュ)を使用
num / value
# 2.0
#numに他の数字を足して新しい数字の入ったnumを生成する
num = num + value
num
# 8
#上記の省略記法は「変数名1 += 変数名2」
num += value
num
# 8
最後の2つは最初はちょっと分かりづらいですが、既存の変数に変数同士の計算の結果を代入するということもプログラミングではよく行います。
そして、後者はnum = num + valueの省略記法で、足し算だけではなく他の四則演算も省略可能です。
四則演算(商の切り下げ、剰余、べき乗)
次は少しだけ複雑な四則演算の計算です。
#四則演算以外
#変数宣言
num = 10
value = 3
#商を計算
num / value
# 3.3333333333333335
#商を切り下げた値を求めるには"//"(スラッシュ2つ)を使用
num // value
# 3
#剰余を求めるには"%"(パーセント)を使用
num % value
# 1
#べき乗を求めるには**(アスタリスク2つ)を使用
num ** value
# 1000
“//"(スラッシュ2つ)について、10 / 3の商は3.33•••となりますので、この商の小数点を切り下げた値は3ですね。
また、"%"(パーセント)については剰余を求めるので10/3の余りは1です。
シフト演算とビット演算
次はシフト演算とビット演算です。
#シフト演算とビット演算
#変数宣言
num = 8
value = 24
#シフト演算は"<<"(左向きの等号2つ)で左にシフトでき、">>"で右(右向きの等号2つ)にシフトできる
#右にシフト
num >> 2
# 2
#左にシフト
num << 1
# 16
#ビット演算
#論理積は"&"(アンド)を使用
num & value
# 8
#論理和は"|"(パイプ)を使用
num | value
# 24
あとは2進数、8進数、16進数、及び複素数の計算方法もあるのですが、ちょっとマニアックなのでここでは割愛します。
以上がPythonで可能な計算とその計算で用いる記号です。
数値型を操作するmathライブラリとメソッド一部紹介
最後に数値型を操作する際に便利なmathライブラリを紹介します。
#mathライブラリのimport
import math
#変数宣言
num = 9
#平方根を求める
math.sqrt(num)
# 3.0
#対数を取る
math.log(num,3)
# 2.0
#math関数の詳細をhelp関数で調べる
help(math)
# Help on module math:
# NAME
# math
# MODULE REFERENCE
# https://docs.python.org/3.8/library/math
# The following documentation is automatically generated from the Python
# source files. It may be incomplete, incorrect or include features that
# are considered implementation detail and may vary between Python
# implementations. When in doubt, consult the module reference at the
# location listed above.
# DESCRIPTION
# This module provides access to the mathematical functions
# defined by the C standard.
# FUNCTIONS
# acos(x, /)
# Return the arc cosine (measured in radians) of x.
# acosh(x, /)
# Return the inverse hyperbolic cosine of x.
# asin(x, /)
# Return the arc sine (measured in radians) of x.
# asinh(x, /)
# Return the inverse hyperbolic sine of x.
# atan(x, /)
# Return the arc tangent (measured in radians) of x.
# atan2(y, x, /)
# Return the arc tangent (measured in radians) of y/x.
# Unlike atan(y/x), the signs of both x and y are considered.
# atanh(x, /)
# Return the inverse hyperbolic tangent of x.
# ceil(x, /)
# Return the ceiling of x as an Integral.
# This is the smallest integer >= x.
# comb(n, k, /)
# Number of ways to choose k items from n items without repetition and without order.
# Evaluates to n! / (k! * (n - k)!) when k <= n and evaluates
# to zero when k > n.
# Also called the binomial coefficient because it is equivalent
# to the coefficient of k-th term in polynomial expansion of the
# expression (1 + x)**n.
# Raises TypeError if either of the arguments are not integers.
# Raises ValueError if either of the arguments are negative.
# copysign(x, y, /)
# Return a float with the magnitude (absolute value) of x but the sign of y.
# On platforms that support signed zeros, copysign(1.0, -0.0)
# returns -1.0.
# cos(x, /)
# Return the cosine of x (measured in radians).
# cosh(x, /)
# Return the hyperbolic cosine of x.
# degrees(x, /)
# Convert angle x from radians to degrees.
# dist(p, q, /)
# Return the Euclidean distance between two points p and q.
# The points should be specified as sequences (or iterables) of
# coordinates. Both inputs must have the same dimension.
# Roughly equivalent to:
# sqrt(sum((px - qx) ** 2.0 for px, qx in zip(p, q)))
# erf(x, /)
# Error function at x.
# erfc(x, /)
# Complementary error function at x.
# exp(x, /)
# Return e raised to the power of x.
# expm1(x, /)
# Return exp(x)-1.
# This function avoids the loss of precision involved in the direct evaluation of exp(x)-1 for small x.
# fabs(x, /)
# Return the absolute value of the float x.
# factorial(x, /)
# Find x!.
# Raise a ValueError if x is negative or non-integral.
# floor(x, /)
# Return the floor of x as an Integral.
# This is the largest integer <= x.
# fmod(x, y, /)
# Return fmod(x, y), according to platform C.
# x % y may differ.
# frexp(x, /)
# Return the mantissa and exponent of x, as pair (m, e).
# m is a float and e is an int, such that x = m * 2.**e.
# If x is 0, m and e are both 0. Else 0.5 <= abs(m) < 1.0.
# fsum(seq, /)
# Return an accurate floating point sum of values in the iterable seq.
# Assumes IEEE-754 floating point arithmetic.
# gamma(x, /)
# Gamma function at x.
# gcd(x, y, /)
# greatest common divisor of x and y
# hypot(...)
# hypot(*coordinates) -> value
# Multidimensional Euclidean distance from the origin to a point.
# Roughly equivalent to:
# sqrt(sum(x**2 for x in coordinates))
# For a two dimensional point (x, y), gives the hypotenuse
# using the Pythagorean theorem: sqrt(x*x + y*y).
# For example, the hypotenuse of a 3/4/5 right triangle is:
# >>> hypot(3.0, 4.0)
# 5.0
# isclose(a, b, *, rel_tol=1e-09, abs_tol=0.0)
# Determine whether two floating point numbers are close in value.
# rel_tol
# maximum difference for being considered "close", relative to the
# magnitude of the input values
# abs_tol
# maximum difference for being considered "close", regardless of the
# magnitude of the input values
# Return True if a is close in value to b, and False otherwise.
# For the values to be considered close, the difference between them
# must be smaller than at least one of the tolerances.
# -inf, inf and NaN behave similarly to the IEEE 754 Standard. That
# is, NaN is not close to anything, even itself. inf and -inf are
# only close to themselves.
# isfinite(x, /)
# Return True if x is neither an infinity nor a NaN, and False otherwise.
# isinf(x, /)
# Return True if x is a positive or negative infinity, and False otherwise.
# isnan(x, /)
# Return True if x is a NaN (not a number), and False otherwise.
# isqrt(n, /)
# Return the integer part of the square root of the input.
# ldexp(x, i, /)
# Return x * (2**i).
# This is essentially the inverse of frexp().
# lgamma(x, /)
# Natural logarithm of absolute value of Gamma function at x.
# log(...)
# log(x, [base=math.e])
# Return the logarithm of x to the given base.
# If the base not specified, returns the natural logarithm (base e) of x.
# log10(x, /)
# Return the base 10 logarithm of x.
# log1p(x, /)
# Return the natural logarithm of 1+x (base e).
# The result is computed in a way which is accurate for x near zero.
# log2(x, /)
# Return the base 2 logarithm of x.
# modf(x, /)
# Return the fractional and integer parts of x.
# Both results carry the sign of x and are floats.
# perm(n, k=None, /)
# Number of ways to choose k items from n items without repetition and with order.
# Evaluates to n! / (n - k)! when k <= n and evaluates
# to zero when k > n.
# If k is not specified or is None, then k defaults to n
# and the function returns n!.
# Raises TypeError if either of the arguments are not integers.
# Raises ValueError if either of the arguments are negative.
# pow(x, y, /)
# Return x**y (x to the power of y).
# prod(iterable, /, *, start=1)
# Calculate the product of all the elements in the input iterable.
# The default start value for the product is 1.
# When the iterable is empty, return the start value. This function is
# intended specifically for use with numeric values and may reject
# non-numeric types.
# radians(x, /)
# Convert angle x from degrees to radians.
# remainder(x, y, /)
# Difference between x and the closest integer multiple of y.
# Return x - n*y where n*y is the closest integer multiple of y.
# In the case where x is exactly halfway between two multiples of
# y, the nearest even value of n is used. The result is always exact.
# sin(x, /)
# Return the sine of x (measured in radians).
# sinh(x, /)
# Return the hyperbolic sine of x.
# sqrt(x, /)
# Return the square root of x.
# tan(x, /)
# Return the tangent of x (measured in radians).
# tanh(x, /)
# Return the hyperbolic tangent of x.
# trunc(x, /)
# Truncates the Real x to the nearest Integral toward 0.
# Uses the __trunc__ magic method.
# DATA
# e = 2.718281828459045
# inf = inf
# nan = nan
# pi = 3.141592653589793
# tau = 6.283185307179586
help(ライブラリ名)とすれば、そのライブラリが持っているメソッドや値を参照できますので覚えておくと便利です。
参考資料
この記事はUdemyのPython+FlaskでのWebアプリケーション開発講座!!~0からFlaskをマスターしてSNSを作成する~という講座と現役シリコンバレーエンジニアが教えるPython 3 入門 + 応用 +アメリカのシリコンバレー流コードスタイルという2つの講座を参考にさせていただき、作成しました。
Python+FlaskでのWebアプリケーション開発講座!!~0からFlaskをマスターしてSNSを作成する~
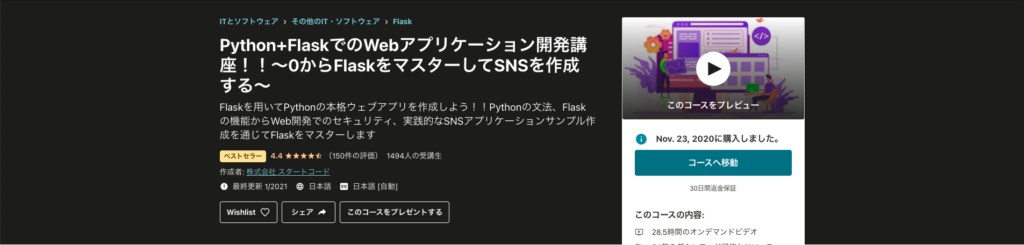
当講座は最低限のWeb開発の知識を知っていることが前提とはなりますが、とにかくPythonの説明とFlask開発の為の説明が充実しているのでおすすめです。
28時間に及ぶ長丁場の講座にはなりますが、絶対に聞く価値がある講座です。
これをマスターすればPythonでどんなアプリケーションでも作ることができると思います。
当講座のおすすめポイントを以下にまとめておきます。
とにかく説明が充実している
Webアプリ開発におけるフレームワークがなぜ有益なのか知ることができる
セキュリティ対策、Ajaxなどの技術も知れる
現役シリコンバレーエンジニアが教えるPython 3 入門 + 応用 +アメリカのシリコンバレー流コードスタイル
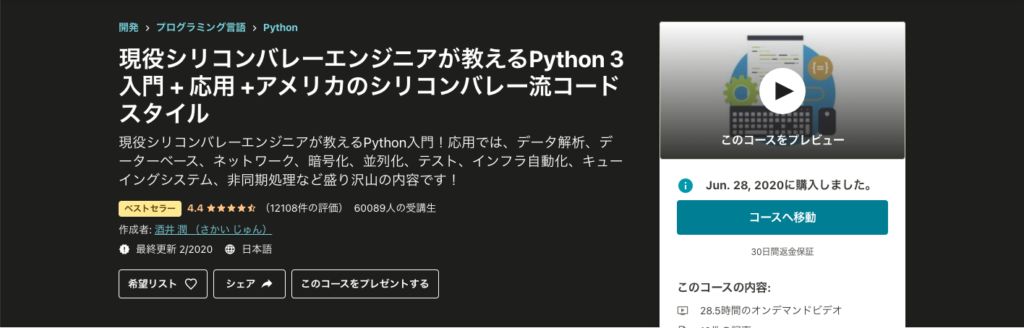
当講座はPythonの基礎から応用まで幅広く学べる講座なのでおすすめです。
この講座の講師はとにかくPythonについての知識が豊富ですし、話も適度な速さで聞き取りやすいです。さすがのシリコンバレーです。
また、最後の方に機械学習で使うライブラリについても解説があるので、データサイエンス・AIについても多少知ることができます。
当講座のおすすめポイントを以下にまとめておきます。
シリコンバレーで働いているということもあり、講師のPythonの知識が豊富
話も適度な速さで聞き取りやすい
コードの意味だけでなく、それをどう応用するかまで解説してくれる
なお、Udemyについては以下の記事でまとめていますのでご参考ください。
まとめ
ということで、今回はPythonの数値型の宣言と数値型の色々な計算方法を解説しました。
数値の計算はプログラミングをする上でよく出てくるテーマなので、ぜひ押さえていただければと思います。
では、今回はここまでとさせていただきます。